Microcontroller software
This page contains assembly-, C and other useful code that I have made for various microcontrollers.
Those include AM186ER (80186 architecture), Motorola MC68HC11 and the ATMega series from
Atmel.
Note that a small part of the sourcecode, especially the MC68HC11 assembler has Danish comments but every variable, label and
function should be in English.
Low-level drivers in C
MAX3100 SPI/Microwire UART driver
A driver for the MAX3100, which I used in a network communication
project using a custom RTOS for AM186ER.
Note: You will not get this to compile as you'll be missing
the includes from the RTOS. Looking over the code, you should
still be able to figure out the buffer handling, interrupt
control and so on.
max3100.h
max3100.c
Field Programmable Analog Array (FPAA) support routines
This contains robust functions to load primary configuration data onto
a FPAA (AN221E04 from Anadigm) using ATMega microcontrollers (fpaa_loadConfig() is the
most important function to consider). A flowchart of the process
can be seen
here.
Note: There are alot of dependencies here which I cannot include
(you will not get it to compile), but you will get a good idea
on how to program the FPAA.
Note: This is dependant on
Procyon AVRLib for low-level routines.
fpaa.h
fpaa.c
DS1305 Serial Alarm Real-Time Clock driver
This is a basic driver for the DS1305 SPI RTC, allowing one
to read and write registers.
Note: This is dependant on
Procyon AVRLib for low-level routines.
ds1305.h
ds1305.c
Simple Dynamic Memory Allocator (malloc) for microcontrollers
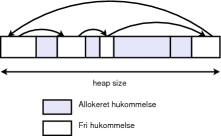
A POSIX-compliant memory allocator using a first-fit strategy
and singly linked-lists for allocating unused space on the heap. Space reserved by malloc() can later be released with free().
The design is optimized for allocation search time and is thus
incompatible with reallocation (ie realloc()).
Note: Part of this code is strongly connected with a specific
RTOS, but you should still be able to reuse the algorithm
and link-structure.
malloc.h
malloc.c
Embedded FAT16/FAT32 filesystem with read/write support
My modified version of the
DOSFS Embedded FAT-Compatible Filesystem written by
Lewin A.R.W Edwards. A fairly lightweight (see above URL) FAT16/32
filesystem with read/write support, to which I had to make some
modifications for reliable functionality on ATMega with GCC.
See the URL above for technical documentation on how to integrate
with your own project.
Note: A few sections of the code where 32-bit
multiplication/division/modulus
is done, there are some critical sections where I have disabled all
interrupts. This was due to a bug in GCC, where R1 would not be
reset to 0 (RISC programs assume this) after an arithmetic operation. This is solved in newer versions so you may remove the critical sections.
Note 2: When formatting a card on Windows, you cannot be sure that
it creates a partition table. This gives a problem when trying to
mount a volume, as the code will have to guess where it starts.
The procedure mountFatVolume() in storage.c has ways to circumvent
this but it is dangerous to rely on.
dosfs.h
dosfs.c
High-level routines:
storage.h
storage.c
MultiMedia and SD Flash Card SPI Interfacing
This is an almost complete rewrite of the MMC/SD SPI interface
routines of
Procyon AVRLib, which I found to be unstable in initializing certain
card types.
This implementation follows Sandisks MMC/SD specification closely
and is rather conservative in regards to waitstates and repetitions
(a flowchart of the initialization can be seen
here)
During tests with a 16MHz ATMega128 microcontroller, it takes around
5 milliseconds to write 512 bytes. This is not a hard limit though,
as the card might not always be able to keep up and delay a write
of up to 10 milliseconds.
mmc.h
mmc.c
Using the PCF8574 I2C I/O extender
This piece of code is for a user-interface board
built around a PCF8574 8-bit I2C I/O extender.
An image of the UI Board connected to the FPAA-based
datalogger
can be seen
here.
uiboard.h
uiboard.c
ATMega specific
Initialization of the A/D converter on ATMega8
Code showing how to initialize the A/D converter on
an ATMega8.
Note: Contains leftovers from some CPLD interfacing, just comment it.
adc.h
adc.c
PWM on ATMega8
Routines for starting the PWM output and setting the dutycycle
on the ATMega8.
pwm.h
pwm.c
SPI on ATMega
This code contains initialization and transfer routines for SPI
on ATMega microcontrollers
spi.h
spi.c
Useful C functions
Curveform generation
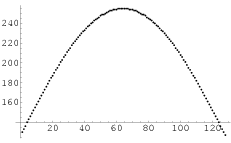
Code to generate square, sawtooth, triangular and sinus
waveforms. The sinus is stored as lookup, with only half of the
waveform in memory (using horizontal symmetry only, could be
improved to using only 1/4th of the waveform).
Note: The frequency control is ATMega-specific.
curve.h
curve.c
Example of commandline interpreter in C
This program for an ATMega8 gives an example of a simple
commandline interpreter with single-character commands.
main.c
Advanced Statemachine Control of a H-bridge
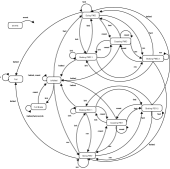
This code implements a Moore-type
statemachine controlling
a H-bridge. It manages the startup, braking and reversing
of a connected DC-motor using RPM-feedback.
The statemachine uses a state transition
table and can thus easily be used and debugged in
other purposes.
h-bridge.h
h-bridge.c
Linear Algebra support routines
This code contains basic linear algebra functions to
support matrix addition, multiplication and substraction
on ATMega microcontrollers (should be easily portable though).
It is based upon a matrix datastructure from
this link.
Note: Don't expect blazing performance, this is really only
meant for initial testing of algorithms.
matrix.h
matrix.c
Control Systems
State-feedback Controller with State estimation
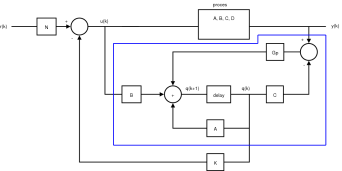
This is an implementation of a State-feedback controller
with estimation of all states.
Though this controller is tailor-fit to a specific
project, you should be able to figure the program flow
of the controller.
statecontrol.h
statecontrol.c
MC68HC11 Assembly
MC68HC11 Assembler for interfacing HD44780A-compatible LCD's
Code for writing lines to a 2-line HD44780A-compatible LCD
controller in 8-bit mode. Based from code by Ib Refer
and Rasmus Maagaard.
It assumes that port B of the HC11 is used as
output with B0-7 mapping to the respective data pins on the
LCD. 3 pins on port A is uesd for control with A3 mapping to
E (LCD-Enable), A4 to R/W (Read/Write) and A5 to RS
(RegisterSelect).
LCD2LIN.ASM
MC68HC11 Keyboard Debouncing in Software
Simple debounce of keys, using busy-wait.
KEYBOARD.ASM
MC68HC11 SPI bit-banging
This code contains an implementation of SPI using
bit-banging.
IPS_ISR.ASM
PC software tests
Keyboard/Mouse activity monitor on Linux
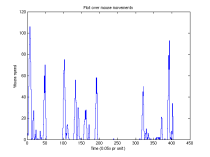
This is a Python script using the Linux kernel event interface
to monitor the activity on input interfaces, mainly a mouse.
It uses the evdev.py interface by Micah Dowty. It was a step
on the way for my Bachelor project, involving analysis
of arm injueries following computer usage.
It is invoked as "./monitor.py /dev/input/eventX", and outputs
to stdout in newline separated text, compatible with tools
such as Matlab/OpenOffice Calc.
monitor.py
evdev.py
evdev.pyc
MAC-address blocking using IPTables
Python script that parses an input file for MAC-addresses and calls iptables to block them.
macparser.py
input.txt - Sample input file